From the moment you tap on an app icon to the instance it closes, a complex series of events takes place behind the scenes. As an Android developer, comprehending the Android lifecycle is crucial for creating robust and seamless applications. Let's delve into this intricate system to understand how your app behaves under different conditions and how you can optimize its performance.
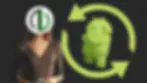
The Basics of Android Lifecycle
At the core of every Android application lies a set of lifecycle methods that dictate the behavior of the app as it transitions between different states. These states include created, started, resumed, paused, stopped, and destroyed. Each of these states plays a vital role in ensuring that your app functions as expected. Let's break down the lifecycle into its fundamental components:
onCreate()
The onCreate() method is where the magic begins. This method is called when the activity is first created, where you can perform initial setup tasks such as inflating layouts, initializing variables, or creating database connections. It is essential to set up your app's initial state in this method to ensure a smooth user experience.
onStart()
After onCreate() comes onStart(). This method signals that the activity is about to become visible to the user. You can perform tasks like registering broadcast receivers or acquiring resources in this phase. It serves as an excellent opportunity to prepare your app for interaction by the user.
onResume()
Next in line is onResume(), indicating that the activity is now in the foreground and ready to receive user input. This phase is crucial for tasks like starting animations, playing audio, or adjusting UI elements. Any functionality that requires user interaction should ideally be placed here.
onPause()
When the user switches to another app or your activity loses focus, the onPause() method is triggered. This stage is where you would pause animations, stop background processes, or release resources to optimize performance. It's essential to handle this method efficiently to ensure a seamless transition for the user.
onStop()
Following onPause() is the onStop() method, signifying that the activity is no longer visible to the user. You can perform tasks like saving user data, releasing CPU-intensive resources, or unregistering listeners in this phase. Proper handling of this method is critical to conserve system resources.
onDestroy()
Finally, we reach onDestroy(), the end of the road for the activity. This method is called before the activity is destroyed, giving you a chance to clean up any resources that are no longer needed. Tasks such as closing database connections, stopping services, or freeing up memory can be performed here.
Handling Configuration Changes
One of the most challenging aspects of the Android lifecycle is dealing with configuration changes such as screen rotations, keyboard availability, or language changes. These events can wreak havoc on your app's state if not handled correctly. Android provides various mechanisms to manage configuration changes efficiently:
onSaveInstanceState()
By overriding the onSaveInstanceState() method, you can save crucial data before an activity is destroyed due to a configuration change. This allows you to restore the activity to its previous state when it is recreated. Storing essential information like user inputs or scroll positions can prevent data loss during these transitions.
onRestoreInstanceState()
After a configuration change, the onRestoreInstanceState() method is called to restore the saved instance state. Here, you can retrieve the data saved in onSaveInstanceState() and reapply it to your activity. This ensures a seamless user experience by retaining the app's previous state across orientation changes.
Managing the Activity Stack
Android applications often involve multiple activities that interact with each other in a hierarchical manner. Understanding how activities are managed in the stack is crucial for maintaining the app's navigation flow and preventing memory leaks. Let's explore some key concepts related to managing the activity stack:
Task and Back Stack
Activities within an app are organized into tasks, with each task containing a back stack of activities. When a new activity is launched, it is added to the top of the stack, and the user can navigate back through the stack by pressing the Back button. Properly managing the back stack is essential for providing a seamless user experience.
Launch Modes
Android offers various launch modes that allow you to control how activities are launched and positioned in the stack. Modes like standard, singleTop, singleTask, and singleInstance provide flexibility in managing the back stack and handling activity transitions. Choosing the appropriate launch mode can streamline your app's navigation flow.
Best Practices for Android Lifecycle Management
Now that we've explored the intricacies of the Android lifecycle, let's discuss some best practices for efficient lifecycle management in your applications. By following these guidelines, you can optimize your app's performance and enhance the user experience:
Separation of Concerns: Divide your app's logic into separate components like Activities, Fragments, and ViewModels to ensure clear responsibilities and maintainable code.
Use LiveData: Utilize LiveData to build reactive UIs that automatically update in response to lifecycle events, ensuring consistency across different states.
Optimize Resource Handling: Release resources in onStop(), unregister listeners in onDestroy(), and implement proper error handling to prevent memory leaks.
Implement ViewModel: Use ViewModel to store and manage UI-related data across configuration changes, ensuring data persistence and a seamless user experience.
Testing: Write comprehensive unit tests for different lifecycle scenarios to validate your app's behavior under various conditions and ensure robustness.
Conclusion
In conclusion, mastering the Android lifecycle is essential for every developer looking to build high-quality applications. By understanding the intricacies of each lifecycle method, managing configuration changes effectively, and implementing best practices, you can create apps that perform optimally and provide a seamless user experience. So, dive deep into the Android lifecycle, harness its power, and elevate your app development skills to new heights!